PROGRAMMING WITH C&C++
PROGRAMMING WITH C&C++ UNIT-2
posted by Madhuri Gongati
Arrays
Arrays are used to store multiple values in a single
variable, instead of declaring separate variables for each value.
To create an array, define the data type (like int) and specify
the name of the array followed by square brackets [].
To insert values to it, use a comma-separated list, inside
curly braces:
int myNumbers[] = {25, 50, 75, 100};
We have now created a variable that holds an array of four
integers.
Access the Elements
of an Array
To access an array element, refer to its index number.
Array indexes start with 0: [0] is the first element. [1] is
the second element, etc.
This statement accesses the value of the first element [0]
in myNumbers:
Example
int myNumbers[] = {25, 50, 75, 100};
printf("%d", myNumbers[0]);
// Outputs 25
Change an Array Element
To change the value of a specific element, refer to the
index number:
Example
myNumbers[0] = 33;
Example
int myNumbers[] = {25, 50, 75, 100};
myNumbers[0] = 33;
printf("%d", myNumbers[0]);
// Now outputs 33 instead of 25
Loop Through an Array
You can loop through the array elements with the for loop.
The following example outputs all elements in the myNumbers
array:
Example
int myNumbers[] = {25, 50, 75, 100};
int i;
for (i = 0; i < 4; i++) {
printf("%d\n", myNumbers[i]);
}
Set Array Size
Another common way to create arrays, is to specify the size
of the array, and add elements later:
Example:1
// Declare an array of four integers:
int myNumbers[4];
// Add elements
myNumbers[0] = 25;
myNumbers[1] = 50;
myNumbers[2] = 75;
myNumbers[3] = 100;
Example:2
Int myNumber[4]={1,2,3,4};
Example:3
Int myNumber[4];
Printf(“enter array elements”);
Scanf(“%d”,&myNumber[i]);
Types of Array in C
There are two types of arrays based on the number of dimensions it has. They are as follows:
- One Dimensional Arrays (1D Array)
- Multidimensional Arrays
1. One Dimensional Array in C
The One-dimensional arrays, also known as 1-D arrays in C are those arrays that have only one dimension.
Syntax of 1D Array in C
datatype array_name [size];
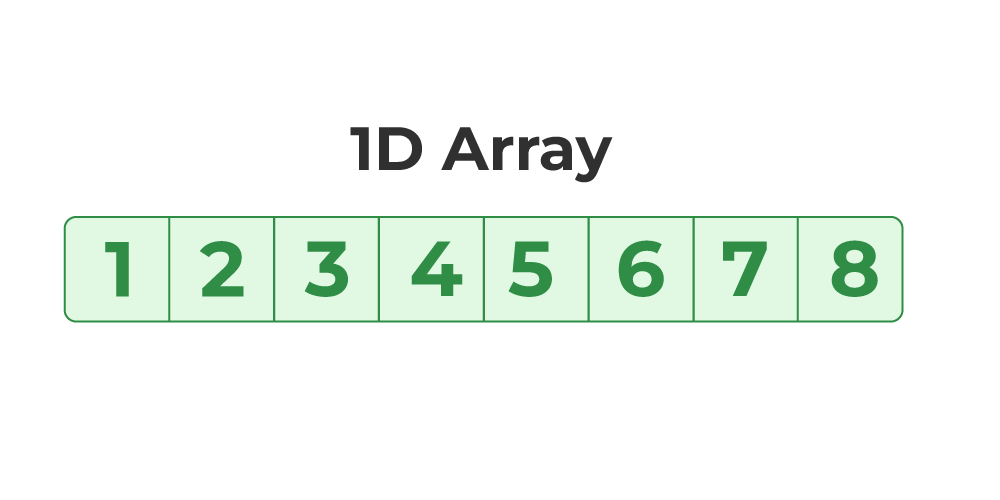
}
2. Multidimensional Array in C
Multi-dimensional Arrays in C are those arrays that have more than one dimension. Some of the popular multidimensional arrays are 2D arrays and 3D arrays. We can declare arrays with more dimensions than 3d arrays but they are avoided as they get very complex and occupy a large amount of space.
A. Two-Dimensional Array in C
A Two-Dimensional array or 2D array in C is an array that has exactly two dimensions. They can be visualized in the form of rows and columns organized in a two-dimensional plane.
Syntax of 2D Array in C
datatype array_name[size1] [size2];
Here,
- size1: Size of the first dimension.
- size2: Size of the second dimension.
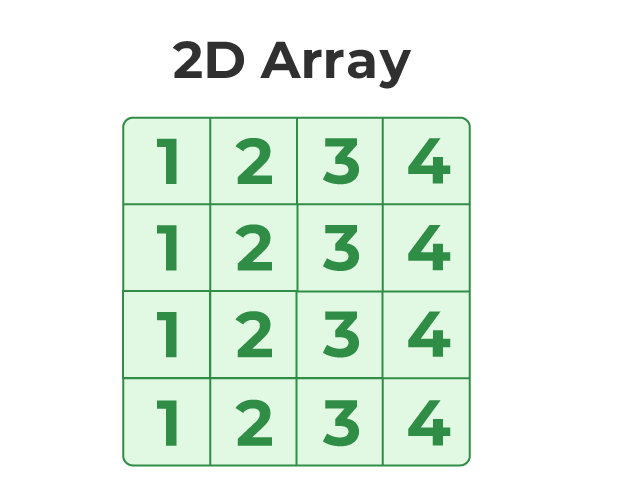
EXAMPLE
Comments
Post a Comment